Acceptance of a string by Pushdown Automaton
Introduction
Before we start with this experiment, we recommend the reader gain an understanding of Determininistic Finite Automata (DFA) and Non-determininistic Finite Automata (DFA).
Question: Over the alphabet {0,1}, Is there a finite state automaton that recognizes the language defined by all strings with equal number of 1's and 0's.
A basic observation is here, we need to keep track of the count of all the 1's and 0's that we have seen, or at least we need to keep track of the imbalance in the count of 1's and 0's that we have seen so far, as we parse the input string. It might seem a little counterintuitive after all the vending machine examples. Sure, we can simulate addition, but we cannot actually “count” entities. The vending machine idea is analogous to counting in a unary alphabet. That is if we had to only keep track of number of 1's that we encountered so far, that is doable. But to recognize the afore mentioned strings, we need something more. Recall that with finite state machines, there’s no associated memory at all, except for what’s available in “control” (current state). Further, there is no history of all the transitions made to reach the current state. It’s almost like a deterministic Markov chain (we have knowledge only of the exact previous state.)
So, how do we count? Could we possibly do this by using some memory? <cue good old computer science style memory- stacks!> Now this new machine, that is a finite state automaton equipped with a stack, is what we call a pushdown automaton.
Pushdown automata is to context-free languages, what NFA’s and DFA’s are to regular languages: PDA’s recognise the languages generated by context-free grammars. Actually, only non-deterministic pushdown automata is truly equivalent in “accepting” power as is the generating power of context-free grammars.
Formal definition
Now that we are familiar with the basics of pushdown automata, here is a formal definition.
A Pushdown automata (PDA for short) can be represented as a 6-tuple , where
- is the set of states
- is the input symbols
- is the stack alphabet/pushdown symbols
- is the transition function that maps into
- is the initial state
- is the set of final states.
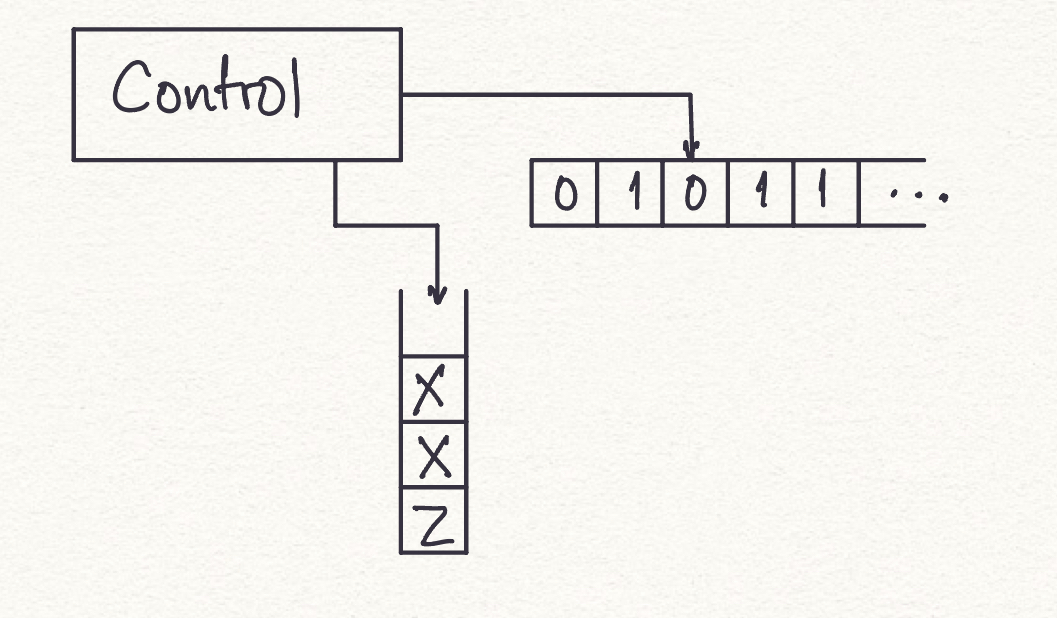
Instantaneous Description shows how a PDA computes an input string and makes a decision to accept it or reject it. It is represented as a triple , where -
- q describes the current state
- w describes the remaining input
- describes the current contents of the stack
A move from one instantaneous description to another is denoted by the symbol . This is known as the turnstile notation.
PDA and Context-Free Languages
Theorem: A language is context-free if and only if there is a pushdown automaton that recognizes it.
This requires the following to be true:
- If a language is context-free, then some PDA recognizes it.
- If a PDA recognizes a language, then the language is context-free.
Examples
The following is a PDA for the language with the set of strings . Here Z (or alternatively ) denotes the empty stack and stack alphabet is .
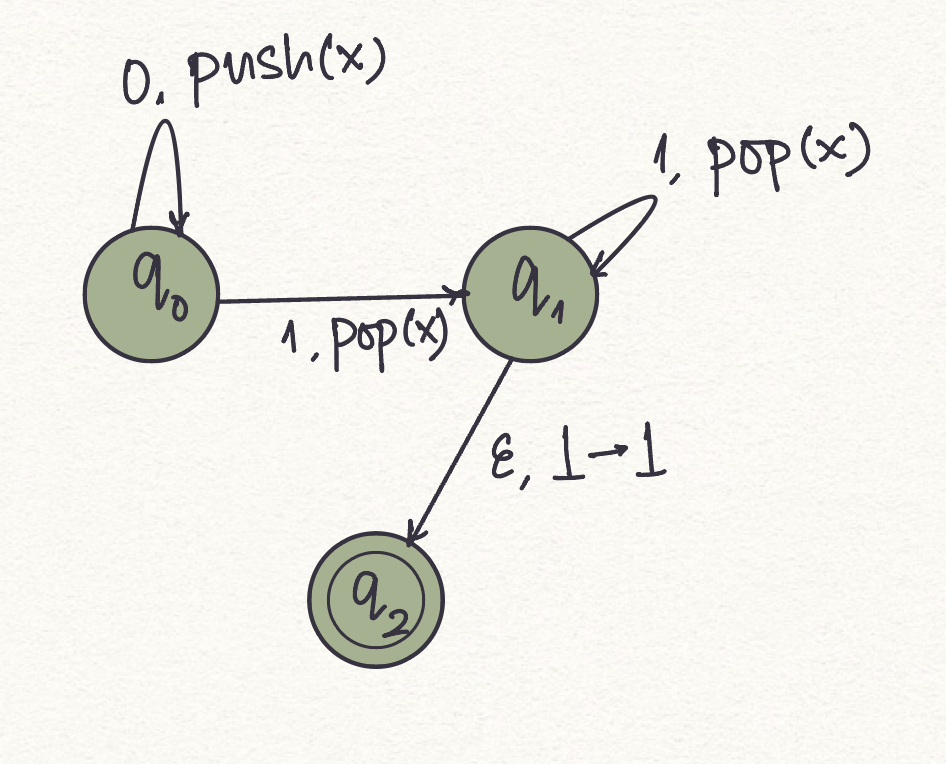
The logic involved in designing this PDA -
- - push an X onto the stack for the first 0 in the input.
- - push an X onto the stack for each subsequent 0 in the input.
- - at the first 1, go to state p and pop one X.
- - Pop an X on each subsequent 1.
- - Bottom of the stack.
Exercise 1: Design a pushdown automaton that accepts a language .
The solution to this builds on the previous example.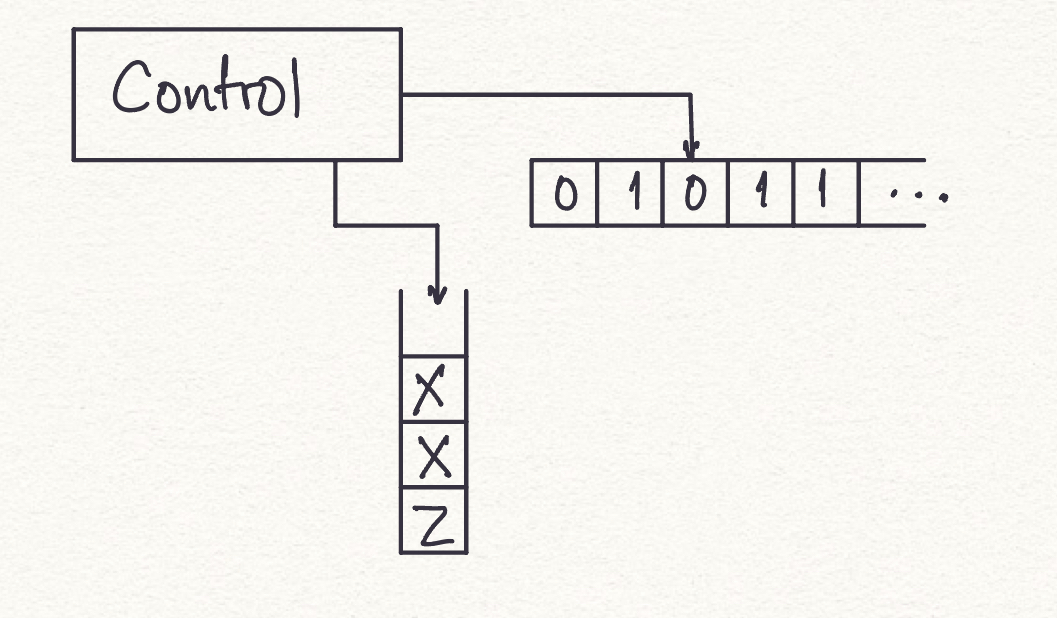
Exercise 2: .
1.
2.
3.
4. table for the transition function
5. is the start state
6.
Exercise 3: Error in LaTeX ' G = { a^i b^j c^k | i, j, k \geq 0; i + j = k\} ': KaTeX parse error: Expected '}', got 'EOF' at end of input: …0; i + j = k\}
1.
2.
3. Error in LaTeX ' \Gamma = \{x, \': KaTeX parse error: Unexpected character: '\' at position 16: \Gamma = \{x, \̲\} $
4. table for the transition function
5. is the start state
6.
Recap
1. What is a pushdown automaton (PDA)?
A pushdown automaton is a finite-state machine with an additional stack that can be used to store and retrieve symbols. It is used to recognize context-free languages.
2. What are the components of a PDA?
A PDA consists of a finite set of states, an input alphabet, a stack alphabet, a transition function, an initial state, a stack symbol, and a set of accepting states.
3. How does a PDA process input?
A PDA reads input symbols one by one and can perform the following actions:
- Read an input symbol and transition to a new state.
- Push a symbol onto the stack.
- Pop a symbol from the stack.
- Read an input symbol and pop a symbol from the stack.
4. What is the role of the stack in a PDA?
The stack in a PDA allows it to remember and match symbols in a last-in, first-out (LIFO) manner. It is used to keep track of information necessary for recognizing context-free languages.
5. What is the difference between a deterministic PDA (DPDA) and a nondeterministic PDA (NPDA)?
In a DPDA, there is at most one transition defined for each input symbol and stack symbol combination. In an NPDA, there can be multiple transitions defined for the same input symbol and stack symbol combination.
6. How is the language recognized by a PDA defined?
The language recognized by a PDA is defined as the set of all strings for which the PDA can reach an accepting state when processing the input.
7. Can a PDA recognize languages that are not context-free?
A7: No, a PDA can only recognize context-free languages. There are languages that are not context-free, and they cannot be recognized by a PDA.8. What is the relationship between PDAs and context-free grammars (CFG)?
PDAs and CFGs are equivalent in terms of language recognition. That is, for every CFG, there exists a PDA that recognizes the same language, and vice versa.9. Can a PDA recognize an infinite language?
Yes, a PDA can recognize infinite languages. The stack allows the PDA to handle potentially unbounded amounts of information and process strings of arbitrary length.10. What are some practical applications of PDAs?
- Programming Languages Parsers: PDAs are widely used in compiler construction to parse and analyze the syntax of programming languages. They help in checking the correctness of the program's structure and generating an Abstract Syntax Tree (AST) for further processing, which has its uses in compilers, linters, and syntax highlighters
- Natural Language Processing: PDAs can be used for syntactic analysis and parsing of natural language sentences. They help in determining the grammatical structure of sentences and identifying the parts of speech, phrases, and dependencies between words. This is essential in tasks such as language understanding, machine translation, and sentiment analysis.
- DNA Sequence Analysis: PDAs are utilized in bioinformatics for analyzing DNA sequences. They can recognize patterns in DNA strings and assist in tasks such as identifying genes, detecting motifs, and predicting protein structures.
Food for Thought
Design a pushdown automaton (PDA) that recognizes the language where w is a string of 0s and 1s .
How about we add another stack? Would that help us solve this problem?