Clipping: Line
Cohen Sutherland's Line Clipping Algorithm
- The Cohen–Sutherland algorithm is a line clipping algorithm used in computer graphics. After dividing a 2D space into 9 regions, the algorithm effectively identifies the lines and line segments that are visible in the viewport, which is the center region of interest.
- The division of regions is based on a window defined by its maximum (xmax, ymax) and minimum (xmin, ymin) coordinates. One region represents the window itself, while the other 8 regions surround it, identified using a 4-digit binary code.
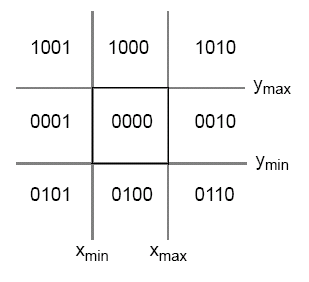
Region Codes Assignment
- Each endpoint of a line segment is assigned a 4-bit binary region code based on its position relative to the clipping window:
- Bit-1 (Top): 1 if y > ymax , 0 otherwise.
- Bit-2 (Bottom): 1 if y < ymin , 0 otherwise.
- Bit-3 (Right): 1 if x > xmax , 0 otherwise.
- Bit-4 (Left): 1 if x < xmin , 0 otherwise.
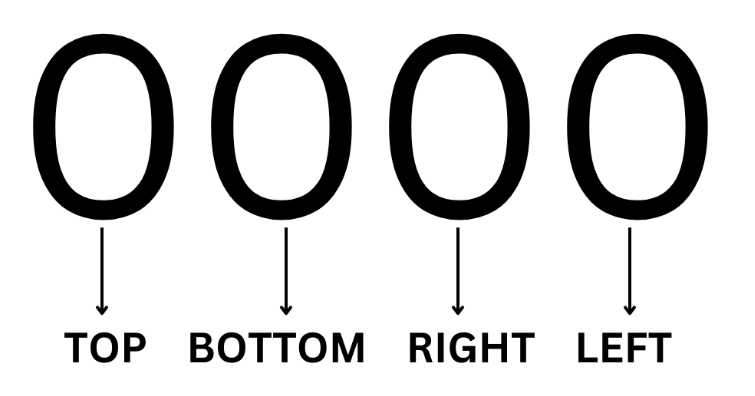
For example:
- If a point (x, y) lies in top-right of the clipping window, its region code will be 1010.
Trivial Acceptance and Rejection:
Trivial Acceptance: When both endpoints have a region code of
0000
, it means both points are inside the clipping window. This is because:- Bit-1 (Top) = 0: Both points are below or at ymax
- Bit-2 (Bottom) = 0: Both points are above or at ymin
- Bit-3 (Right) = 0: Both points are to the left or at xmax
- Bit-4 (Left) = 0: Both points are to the right or at xmin Therefore, the entire line segment must lie within the window.
Trivial Rejection: When the bitwise AND of both endpoint codes is not
0000
, it means both points lie in regions that are completely outside the window. This is because:- If any bit position has 1 in both codes, both points are on the same side of the window (both above, both below, both left, or both right)
- For example, if both points have bit-1 = 1, they are both above the window
- Therefore, the line segment cannot intersect the window and can be rejected
Derivation of Intersection Point Equations
When a line segment needs to be clipped, we need to find its intersection points with the window boundaries. Let's derive these equations:
Line Equation: For a line segment from (x1, y1) to (x2, y2):
- Slope m = (y2 - y1) / (x2 - x1)
- Point-slope form: y - y1 = m(x - x1)
Intersection with Horizontal Boundaries:
For y = ymax:
- Substitute y = ymax in point-slope form
- ymax - y1 = m(x - x1)
- x = x1 + (1/m)(ymax - y1)
For y = ymin:
- Substitute y = ymin in point-slope form
- ymin - y1 = m(x - x1)
- x = x1 + (1/m)(ymin - y1)
Intersection with Vertical Boundaries:
For x = xmax:
- Substitute x = xmax in point-slope form
- y - y1 = m(xmax - x1)
- y = y1 + m(xmax - x1)
For x = xmin:
- Substitute x = xmin in point-slope form
- y - y1 = m(xmin - x1)
- y = y1 + m(xmin - x1)
Line Clipping Algorithm Pseudo Code
Assign region codes to both endpoints points (A(x1, y1) and B(x2, y2)) of the line segment.
Perform a bitwise OR operation on both endpoints:
- If OR == 0000,
- The line is completely inside the window (Trivially accepted).
- Else,
- Perform a bitwise AND operation on both endpoints:
- If AND != 0000,
- The line is not inside the window, it cannot be clipped (Trivially rejected).
- Else,
- The line is partially inside the window and can be considered for clipping.
- If AND != 0000,
- Perform a bitwise AND operation on both endpoints:
- If OR == 0000,
After confirming the line is partially inside the window:
- Find the intersection with the boundary of the window:
- Calculate the slope of the line: m = (y2 - y1) / (x2 - x1).
- Determine the intersection point based on the region code:
- If the line intersects with the top boundary:
- x = x1 + (1 / m) * (ymax - y1)
- Update the intersection point (x, ymax)
- If the line intersects with the bottom boundary:
- x = x1 + (1 / m) * (ymin - y1)
- Update the intersection point (x, ymin)
- If the line intersects with the left boundary:
- y = y1 + m * (xmin - x1)
- Update the intersection point (xmin, y)
- If the line intersects with the right boundary:
- y = y1 + m * (xmax - x1)
- Update the intersection point (xmax, y)
- If the line intersects with the top boundary:
- Find the intersection with the boundary of the window:
Overwrite the endpoint with the new intersection point and update its region code.
Repeat step 2-4 until a trivial accept or reject occurs.
Repeat the entire process for other lines as needed.
- This pseudo code outlines the steps of the Cohen–Sutherland line clipping algorithm, detailing how line segments are processed and clipped against a defined clipping window in 2D space.